Discord Web Hooks are easier than you’d think. It uses a regular `HTTP` web request .
Step1: Creating your webhook url
Go to: Server Settings -> Integrations -> View Webhooks
Then click create webhook.
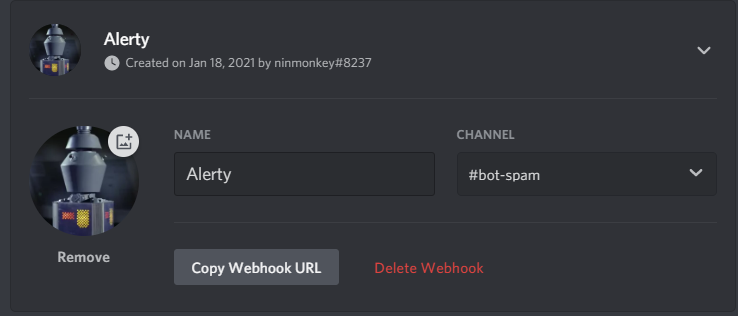
copy webhook url
Step2: Invoke-RestMethod
$webhookUri = 'https://discord.com/api/YourWebhookUrlHere'
$Body = @{
'username' = 'Nomad'
'content' = 'https://memory-alpha.fandom.com/wiki/Nomad'
}
Invoke-RestMethod -Uri $webhookUri -Method 'post' -Body $Body
Success!
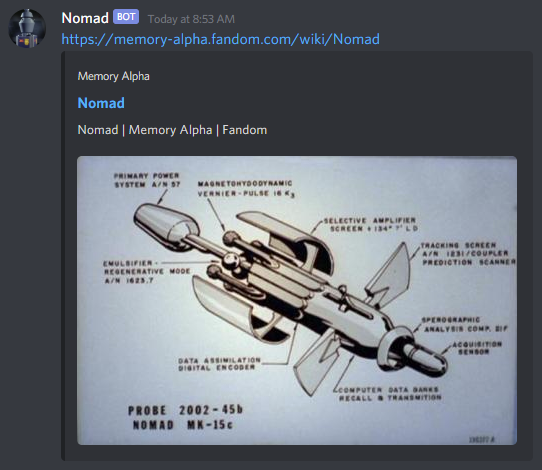
Docs
There are optional webhook arguments to customize the output: https://discord.com/developers/docs/resources/webhook#execute-webhook
Comparison with curl
I tried making curl
easier to read by splitting content, and pipe so that you don’t have to manually escape Json
$Json = @{ 'username' = 'Nomad'; 'content' = 'https://memory-alpha.fandom.com/wiki/Nomad' } | ConvertTo-Json -Compress
$Json | curl -X POST -H 'Content-Type: application/json' -d '@-' $webhookUri